Newton's Version Of Kepler's Third Law Calculator
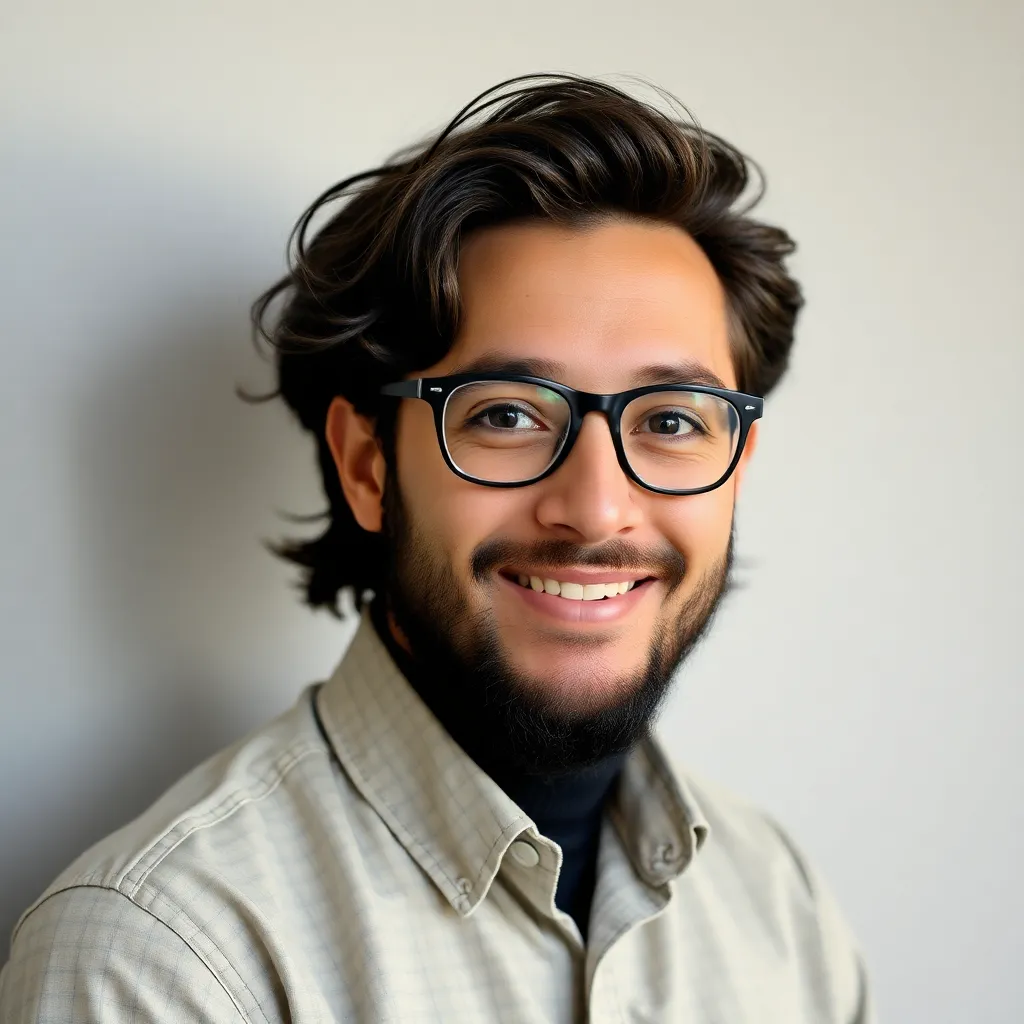
listenit
Apr 22, 2025 · 6 min read

Table of Contents
Newton's Version of Kepler's Third Law Calculator: A Deep Dive
Kepler's Third Law, a cornerstone of celestial mechanics, describes the relationship between the orbital period and the semi-major axis of a planet's orbit around the Sun. While Kepler's original formulation focused on the Sun's gravitational dominance, Newton's version generalized this law to encompass any two bodies orbiting each other under the influence of mutual gravity. This generalization is incredibly powerful, allowing us to calculate orbital parameters for a wide variety of celestial systems, from planets around stars to binary stars orbiting each other. This article will delve into Newton's version of Kepler's Third Law, its mathematical formulation, and its applications, culminating in a conceptual outline for creating a calculator to implement this law.
Understanding Kepler's Third Law: A Brief Recap
Kepler's Third Law, in its simplest form, states that the square of the orbital period of a planet is directly proportional to the cube of the semi-major axis of its orbit. Mathematically, this can be expressed as:
T² ∝ a³
where:
- T represents the orbital period (typically measured in years)
- a represents the semi-major axis of the elliptical orbit (typically measured in astronomical units, AU)
This law holds true for planets orbiting the Sun, but it lacks the underlying physics to explain why this relationship exists.
Newton's Generalization: Introducing Mass
Newton's Law of Universal Gravitation provided the missing piece. He showed that the force of gravity between two objects is directly proportional to the product of their masses and inversely proportional to the square of the distance between their centers. By incorporating this into Kepler's Third Law, Newton arrived at a more comprehensive and universally applicable version:
T² = (4π²/G(M₁ + M₂)) * a³
where:
- T is the orbital period
- a is the semi-major axis
- G is the gravitational constant (approximately 6.674 x 10⁻¹¹ N⋅m²/kg²)
- M₁ and M₂ are the masses of the two orbiting bodies
This equation is significantly more powerful than Kepler's original formulation because it explicitly includes the masses of both objects. This allows us to calculate orbital parameters for systems where the masses of the orbiting bodies are comparable, such as binary star systems or even satellite orbits around planets.
Implications of Newton's Version
The inclusion of mass in Newton's version highlights several crucial aspects:
- Mutual gravitation: Kepler's Law implicitly assumes the Sun's mass is vastly greater than the planet's mass, making the planet's contribution negligible. Newton's version acknowledges the mutual gravitational pull between both bodies.
- Binary systems: This allows us to analyze the dynamics of binary star systems, where both stars have comparable masses and significantly influence each other's orbits.
- Satellite orbits: It allows accurate calculations of satellite orbital periods around planets, considering the planet's mass.
- Exoplanet detection: By observing the wobble of a star caused by an orbiting exoplanet, astronomers can estimate the exoplanet's mass and orbital parameters using Newton's version of Kepler's Third Law.
Building a Newton's Version of Kepler's Third Law Calculator: A Conceptual Outline
Creating a calculator to implement Newton's version of Kepler's Third Law requires a methodical approach. The following outlines the key steps and considerations:
1. Choosing a Programming Language
The choice of programming language depends on your familiarity and the desired level of complexity. Languages like Python, with its rich scientific libraries (NumPy, SciPy), are well-suited for this task. JavaScript could also be used for a web-based calculator.
2. User Interface Design
A user-friendly interface is crucial. The calculator should have clear input fields for:
- Semi-major axis (a): Allowing input in various units (e.g., meters, kilometers, AU) with appropriate unit conversion capabilities.
- Mass of object 1 (M₁): Again, allowing input in various units (e.g., kilograms, solar masses) with unit conversion.
- Mass of object 2 (M₂): Similar to M₁.
- Orbital period (T): Input in various units (e.g., seconds, days, years) with unit conversion.
The calculator should clearly indicate which input values are required and which are calculated. The output should display the calculated value along with the appropriate units. Error handling should be incorporated to manage invalid inputs (e.g., negative values).
3. Implementing the Calculation
The core of the calculator involves implementing Newton's generalized Kepler's Third Law:
import math
def calculate_orbital_period(a, M1, M2, G=6.674e-11):
"""Calculates orbital period using Newton's version of Kepler's Third Law."""
# Unit Conversion (If necessary, add unit conversion functions here)
# ...
T_squared = (4 * math.pi**2 / (G * (M1 + M2))) * a**3
T = math.sqrt(T_squared)
return T
def calculate_semi_major_axis(T, M1, M2, G=6.674e-11):
"""Calculates semi-major axis using Newton's version of Kepler's Third Law."""
#Unit Conversion (If necessary, add unit conversion functions here)
# ...
a_cubed = (G * (M1 + M2) * T**2) / (4 * math.pi**2)
a = a_cubed**(1/3)
return a
# Example usage:
a = 1.496e11 #meters (approx. 1 AU)
M1 = 1.989e30 #kg (mass of Sun)
M2 = 5.972e24 #kg (mass of Earth)
T = calculate_orbital_period(a, M1, M2)
print(f"Orbital Period: {T/ (60*60*24*365.25)} years") #convert to years
T_years = 1 #year
a_calc = calculate_semi_major_axis(T_years * 60*60*24*365.25,M1,M2)
print(f"Semi-major axis: {a_calc/ 1.496e11} AU") #convert to AU
This Python code demonstrates the core calculation. Error handling and unit conversion would need to be added for a robust calculator.
4. Unit Conversion
The calculator needs to handle various input units for mass, distance, and time. This requires implementing functions to convert between different units (e.g., meters to kilometers, kilograms to solar masses, seconds to years).
5. Testing and Validation
Thorough testing is essential to ensure the calculator's accuracy. This involves testing with known values and comparing the results with expected values. The calculator should also handle edge cases and invalid inputs gracefully.
6. User Documentation and Help
Clear documentation and instructions on how to use the calculator are crucial. This includes explaining the input parameters, the output, and handling units.
Advanced Features (Optional)
For a more advanced calculator, you could consider:
- Graphical representation: Visualizing the orbit using a graph.
- Support for different orbital shapes: The current formulation assumes a circular orbit. Adapting it for elliptical orbits would require using the semi-major axis correctly.
- Inclusion of eccentricity: Adding eccentricity as an input parameter would allow calculation for elliptical orbits.
- Integration with astronomical databases: Fetching mass and orbital data from online astronomical databases.
Conclusion
Newton's generalization of Kepler's Third Law is a powerful tool for understanding and calculating the orbital dynamics of celestial bodies. Building a calculator to implement this law provides a practical application of this fundamental principle of physics. By carefully designing the user interface, implementing the calculation correctly, and incorporating error handling and unit conversion, you can create a valuable tool for students, researchers, and anyone interested in exploring the wonders of celestial mechanics. Remember to thoroughly test and validate your calculator to ensure its accuracy and reliability. The journey of creating this calculator will not only enhance your programming skills but also deepen your understanding of a fundamental law governing the universe.
Latest Posts
Latest Posts
-
Do Noble Gases Have Ionization Energy
Apr 22, 2025
-
Is Bromine A Metal Or Nonmetal
Apr 22, 2025
-
Difference Between Molecular And Structural Formula
Apr 22, 2025
-
What Is 2 2 3 As An Improper Fraction
Apr 22, 2025
-
How Many 1 3 Are In A Cup
Apr 22, 2025
Related Post
Thank you for visiting our website which covers about Newton's Version Of Kepler's Third Law Calculator . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.